A magazine where the digital world meets the real world.
On the web
- Home
- Browse by date
- Browse by topic
- Enter the maze
- Follow our blog
- Follow us on Twitter
- Resources for teachers
- Subscribe
In print
What is cs4fn?
- About us
- Contact us
- Partners
- Privacy and cookies
- Copyright and contributions
- Links to other fun sites
- Complete our questionnaire, give us feedback
Search:
The Abracadabra program explained
by Paul Curzon, Queen Mary University of London
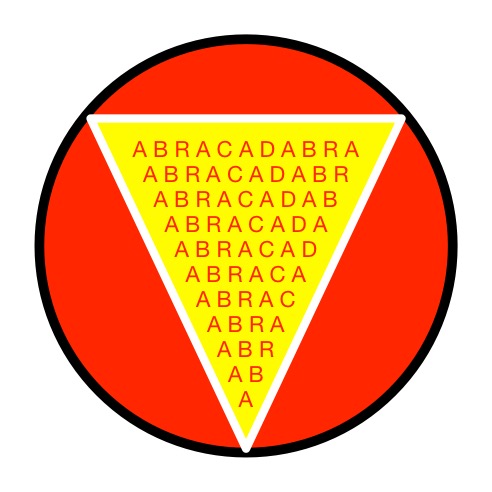
How does our abracadabra program work? Let's go through it a step at a time.
First we are going to need to be able to print individual letters, but each followed by a space. Python has a print command that prints things.
print(letter)
will print the given letter (whatever is stored in variable letter).
However, print follows the letter by a new line (i.e., moves to the start of the next line). Here we want all the letters of a word on one line. We can specify that the end letter is a space rather than a newline like this:
print(letter, end=" ")
We can now make that into our own reusable method called printletter, that will always print a given letter in exactly this way.
def printletter(letter): """Print a single letter followed by a space and no newline""" print(letter, end=" ")
Next we want to print a whole word like this - given the word, print a letter at a time exactly as above. We use a for loop to scan down the letters in the word, passing each in turn to printletter before moving on to the next. Python's for loop can do this. It extracts a letter at a time, storing it in variable, letter to pass to our printletter method.
for letter in word: printletter(letter)
Once the word is printed we do want to move to the next line to start the next word. We can do that with a simple print statement, that prints nothing. We execute it after the loop has completed - so the newline is added only once at the endof the word.
print()
Again we can embed all this in a method, that this time prints a line.
def printline(word): """Print a word out with spaces between each letter""" for letter in word: printletter(letter) print()
Next we need a method to print a given word in a triangle that uses the above method to print each line. This is essentially a for loop again. This time the loop takes a slice of the word from the start up to the current length of the word. We need the for loop to start at the word's length, stop at 0 and subtract 1 each time, before doing it again. We store the length of each line (ie word slice) in variable, currentend. The method range, just creates a list of all the line lengths for the for loop to work its way down.
for currentend in range(len(word), 0, -1):
Each time this for loop takes another slice, it needs to use our printline method to print the supplied word from 0 to the current end.
for currentend in range(len(word), 0, -1): printline(word[0:currentend])
However, this starts each word at the start of the line. We need to add spaces on the front to get the kind of triangle we want. The first line is printed without any spaces, then we want to add an extra space on the front each time. We can do this by creating an accumulator variable (spaces) that we add an extra space to each time round the loop.
Outside the loop we set this accumulator, spaces, to an empty string. Inside the loop we print the current spaces, and at the end of the loop add an extra space for next time round. When we print the spaces we want neither a new line nor an extra space added so specify that printing ends with an empty string.
spaces = "" for currentend in range(len(word), 0, -1): print(spaces, end="") printline(word[0:currentend]) spaces = spaces + " "
Packaging this up we get a method that can print any given word in a triangular shape. The word to be printed is passed to the method and stored in variable, word.
def triangle(word): """Print a given word in a magic triangle removing the last letter each time it is written""" spaces = "" for currentend in range(len(word), 0, -1): print(spaces, end="") printline(word[0:currentend]) spaces = spaces + " "
Now all we need to do is to set it to work on the word "ABRCADABRA" calling our method triangle with the string "ABRACADABRA".
def abracadabra(): """Print ABRACADABRA in a magic triangle""" triangle("ABRACADABRA")
Finally we can call our new method abracadabra() any time we want to create the magic shape with ABRACADABRA.
abracadabra()
Why not invent your own spell words that need to be written in particular patterns, then write programs that write them exactly right every time.